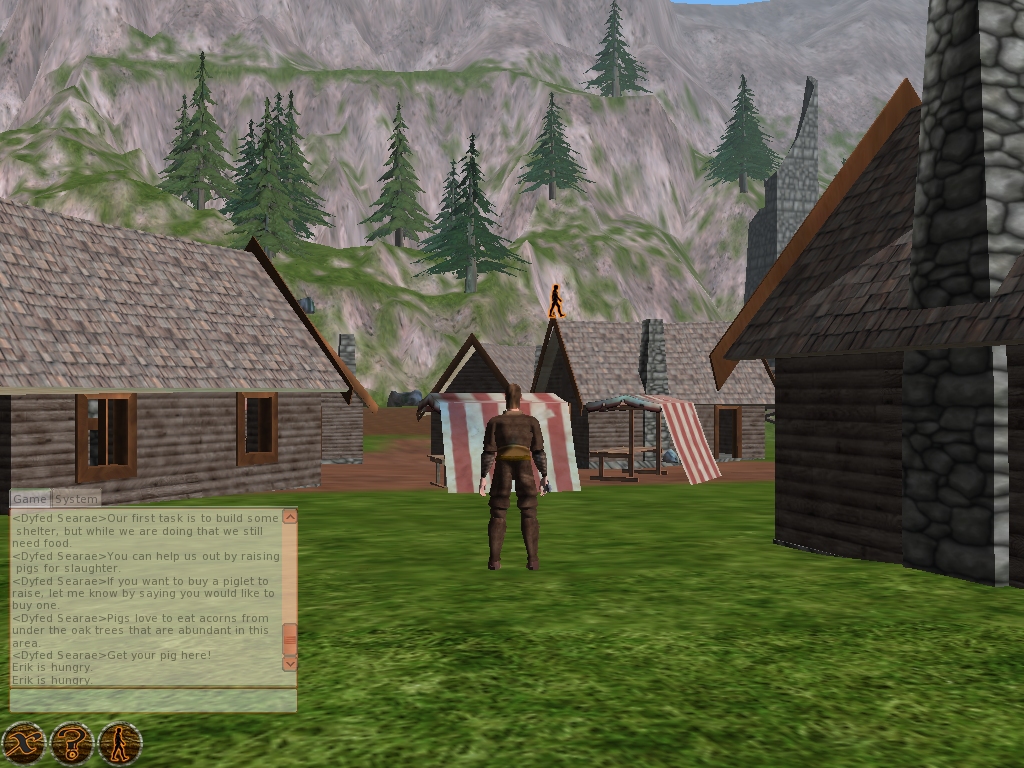
I've been spending the last couple of days finding and fixing smaller bugs in the Ember codebase. The client itself is pretty solid, I very rarely get crashes, and when I do I can most often fix the underlying problem right away. But then there's the issue of shutdown... It's so much easier to not clean up and instead just exit the application, because it doesn't really matter if there's a segfault when shutting down; the memory is about to be reclaimed anyway, right? Well, there's the problem with sockets and other data structures that aren't that nicely shut down when the app crashes. And there's the issue with shutting down partially and then restarting, like disconnecting from a server and the reconnecting. And so on...
As I wrote in this entry I started Ember as a project to teach me C++, so initially I didn't care much about proper resource handling, because there were so many other things that I had to wrap my head around. But not so any more. Which means that I have to fix all of those lingering problems with resources not being released as they should. The most common culprit is Ogre, CEGUI and Lua. The interdependencies of these libraries forces me to be very careful about how I both acquire resources and release them.
I'm thinking it's about time 0.5.0 was released. The thing is that I always dread doing releases because it means I have to put development on hold for usually more than a week while I try to package Ember for both Linux and Windows. The latter one is always the most time consuming, since I do all development on Linux and then port to Windows at release time. So far I've used gcc and the automake toolchain for Linux and Visual Studio for Windows. This means however that I always have to update the Visual Studio project every time I do a release, and more worrying; that I have to work around all the missing features in msvc (compared to gcc). I'm thinking that it might perhaps be better to set up a mingw system on Windows so I can use gcc for both platforms. If I can get the autotools to work on Windows that would be even more excellent.